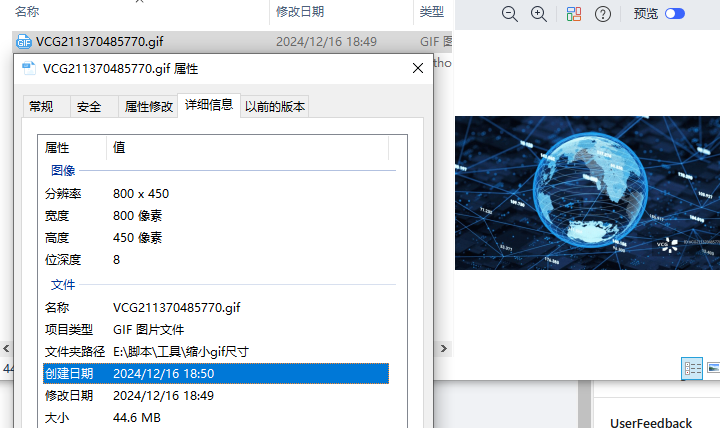
比如我们有一张大小44.6M的动图,想要压缩它。
但是有的网站对压缩的GIF有尺寸上限规定。
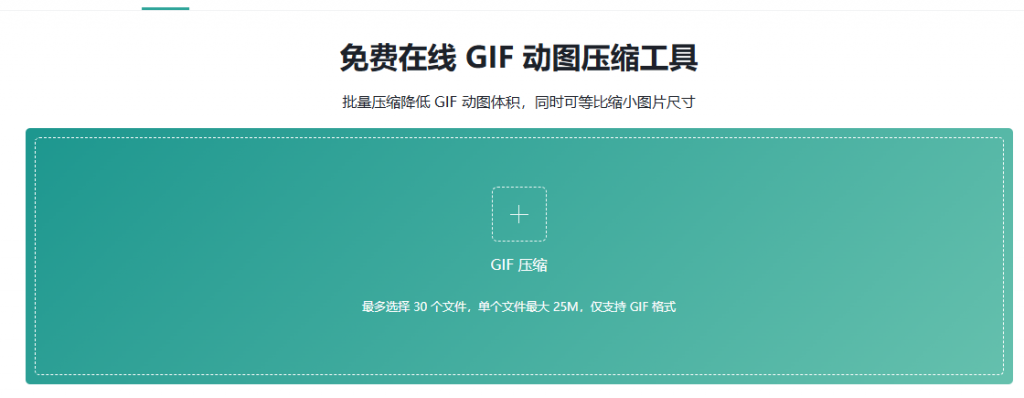
而有一些GIF已经压缩到极致,无法再压缩了。如果用普通网站压缩的方法,可能压缩完之后文件不变小反而变大。这时候就只能修改图片的长宽来压缩图片了。我们指定一个尺寸,然后逐帧替换等比例画面,就可以压缩图片尺寸了,效果如下:
附上完整代码:
import os
from PIL import Image, ImageSequence
def resize_gif(input_path, output_path, new_size):
# 打开GIF文件
gif = Image.open(input_path)
# 获取GIF的原始尺寸
original_size = gif.size
# 计算新的尺寸,保持比例
width, height = new_size
if width is None:
width = int(height * original_size[0] / original_size[1])
if height is None:
height = int(width * original_size[1] / original_size[0])
# 创建一个新的GIF帧列表
frames = []
# 遍历GIF的每一帧
for frame in ImageSequence.Iterator(gif):
# 调整每一帧的尺寸
resized_frame = frame.resize((width, height), Image.Resampling.LANCZOS)
frames.append(resized_frame)
# 保存调整后的GIF
if len(frames) == 1:
frames[0].save(output_path)
else:
frames[0].save(output_path, save_all=True, append_images=frames[1:], loop=0, duration=gif.info['duration'])
# 遍历当前文件夹中的所有GIF文件
current_directory = os.getcwd()
gif_files = [f for f in os.listdir(current_directory) if f.endswith('.gif')]
# 处理每个GIF文件
for gif_file in gif_files:
input_gif = os.path.join(current_directory, gif_file)
output_gif = os.path.join(current_directory, f"resized_{gif_file}")
new_size = (320, None) # 宽度为320,高度按比例调整
resize_gif(input_gif, output_gif, new_size)